So you’ve got some open source C# code, and you want to publish it for other users in Unity? I’ve explored a few options, here are the pros and cons.
Continue readingunity
VoronatorSharp
I’ve relased a new library, VoronatorSharp.
VoronatorSharp is a C# library that computes Voronoi diagrams. The Voronoi diagram for a collection of points is the polygons that enclose the areas nearest each of those sites.
Voronoi diagrams have applications in a number of areas such as computer graphics.
This library features:
- Computes Voronoi diagrams and Delaunay triangulations.
- Voronoi polygons can be clipped to a rectangular area.
- Uses a
n log(n)
sweephull algorithm. - The implementation attempts to minimize memory allocations.
- Integrates with Unity or can be be used standalone.
- Uses robust orientation code.
- Handles Voronoi diagrams with only 1 or 2 points, and collinear points.
Mosaic Paint
As a side-project of a side-project, I’ve made a little painting program. It’s like a normal paint program, only you paint on a tile grid instead of pixels. You can create mosaic and stained-glass style images.
Continue readingVaria 1.0 released
Announcing Varia, a new Unity asset for adding small random variations into your games.
Spice up your levels, remix your gameplay, and make your assets go further for you. It’s FREE!
Using Unity’s TextGenerator.verts
TextGenerator.verts
is meant to give the position information of every character in a given string. This is useful in Unity if you need to align something with exactly where some particular text is occuring, if for some reason you are not already using TextMeshPro.
Older Unity versions created 4 verts for every character, which made life easy. But now many non-rendering characters don’t have verts generated for them, and the relationship between verts and characters is undocumented. I’ve reverse engineered it, as best as I can tell:
int? GetVertForPosition(int position, string text, TextGenerator textGenerator)
{
var c = 0;
var vert = 0;
for (var i = 0; i < position; i++)
{
if (textGenerator.characters.Count <= c)
return null;
if (!char.IsWhiteSpace(text[i]) && textGenerator.characters[c].charWidth > 0)
vert += 4;
if (text[i] != '\n')
c++;
}
return vert;
}
Junkbot Arena
Lucky Fluke
Tessera Pro Released
Due to demand, I’ve now released Tessera Pro, an upgraded version of Tessera which comes with source code and some extra features. Hopefully with more features to come.
I’m really pleased with how the project has been recieved so far, and am hoping to see some awesome games made with it.
Check it out on the Unity asset store.
Messing with Unity’s GUIDs
I recently released an addon in the Unity asset store. It’s actually two addons: Tessera Pro is a fully featured copy, with complete source code, and Tessera which has cut down features, and you just get a precompiled .dll.
I quickly discovered a big problem – if you upgrade from Tessera to Tessera Pro, then all your scenes become broken. You get this error, which is likely familiar to veteran Unity developers.
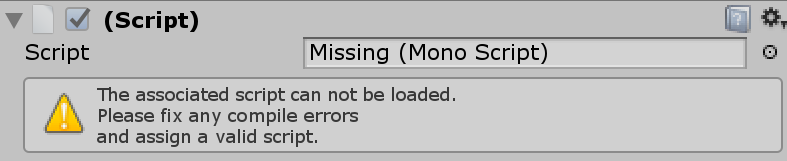
I’ll go into what’s happening in general, and how I dealt with it.
Continue readingTessera 3d tile level generation
After a lot of work, I’ve prepared my first ever commercial release. It’s an addon for Unity that lets you procedurally generate levels and other things based on a simple tile setup.